Basic guide
This guide will describe how you can setup Kubb + use the TypeScript plugin to generate types based on the petStore.yaml
file.
The setup will contain from the beginning the following folder structure
typescript
.
├── src
├── petStore.yaml
├── kubb.config.js
└── package.json
.
├── src
├── petStore.yaml
├── kubb.config.js
└── package.json
Step one
Setup your kubb.config.js
file based on the Quick-start.
We will add here the Swagger and SwaggerTypescript(which is depended on the Swagger plugin) plugin, those 2 plugins together will generate the TypeScript types.
- Next to that we will also set
output
to false for the Swagger plugin because we don't need the plugin to generate the JSON schemas for us. - For the SwaggerTypescript plugin we will set the
output
to the models folder.
typescript
import { defineConfig } from '@kubb/core'
import createSwagger from '@kubb/swagger'
import createSwaggerTS from '@kubb/swagger-ts'
export default defineConfig(async () => {
return {
root: '.',
input: {
path: './petStore.yaml',
},
output: {
path: './src',
},
plugins: [createSwagger({ output: false, validate: true }), createSwaggerTS({ output: 'models' })],
}
})
import { defineConfig } from '@kubb/core'
import createSwagger from '@kubb/swagger'
import createSwaggerTS from '@kubb/swagger-ts'
export default defineConfig(async () => {
return {
root: '.',
input: {
path: './petStore.yaml',
},
output: {
path: './src',
},
plugins: [createSwagger({ output: false, validate: true }), createSwaggerTS({ output: 'models' })],
}
})
This will result in the following folder structure when Kubb has been executed
typescript
.
├── src/
│ └── models/
│ ├── typeA.ts
│ └── typeB.ts
├── petStore.yaml
├── kubb.config.js
└── package.json
.
├── src/
│ └── models/
│ ├── typeA.ts
│ └── typeB.ts
├── petStore.yaml
├── kubb.config.js
└── package.json
Step two
Update your package.json
and install Kubb
, see installation.
Your package.json
will look like this:
json
{
"name": "your project",
"scripts": {
"generate": "kubb generate --config kubb.config.js"
},
"dependencies": {
"@kubb/cli": "latest",
"@kubb/core": "latest",
"@kubb/swagger": "latest",
"@kubb/swagger-ts": "latest"
}
}
{
"name": "your project",
"scripts": {
"generate": "kubb generate --config kubb.config.js"
},
"dependencies": {
"@kubb/cli": "latest",
"@kubb/core": "latest",
"@kubb/swagger": "latest",
"@kubb/swagger-ts": "latest"
}
}
Step three
Run the Kubb script with the following command.
shell
bun run generate
bun run generate
shell
pnpm run generate
pnpm run generate
shell
npm run generate
npm run generate
shell
yarn run generate
yarn run generate
Step four
Drink a 🍺 and watch Kubb generate your files.
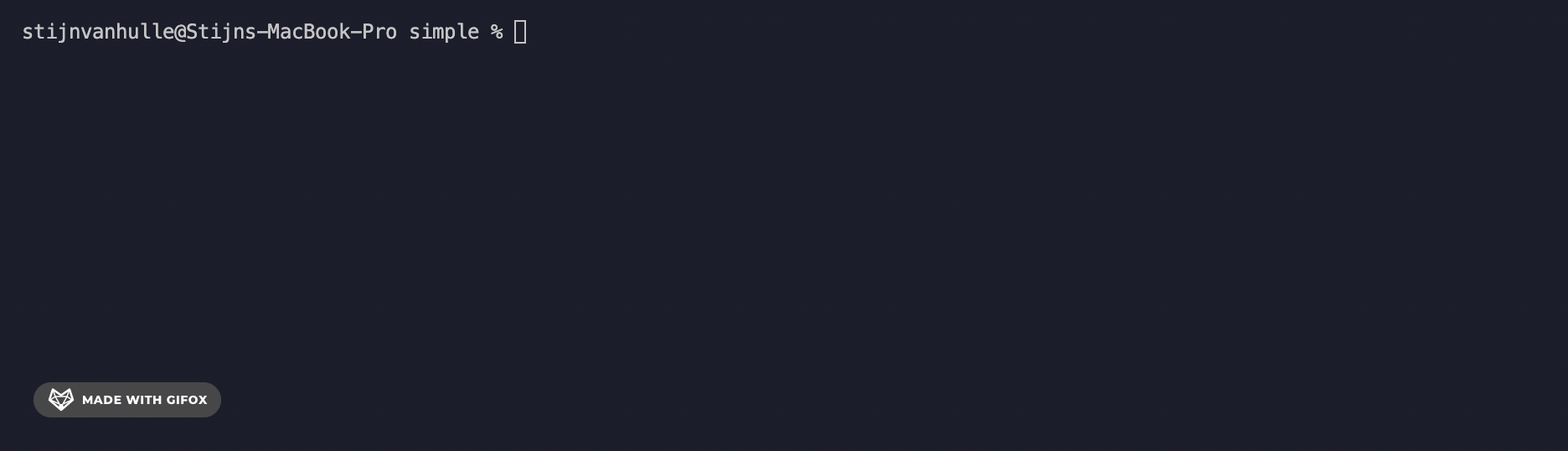